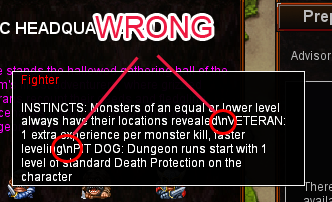
So here’s a fun fact: Managing text in games is a massive headache. There are a number of problems that you are likely to encounter, they start out small, and then grow over time. First you just have the text you want displayed sitting in the code files … but then you realise you might need to localise your game for other countries, so you make some changes. Soon you have a TextManager class, holding all your text. Each piece of text is allocated with a key, and you only reference the keys in code. This way, when you need to translate, you can just have a translator go through all the keys and you don’t have to change any of the code! Brilliant!
Then the trouble starts.
Your text library has gotten so large that it can’t be paged around memory properly, and causes weird and wonderful crashes. So you split it into two .. no three … wait … four dictionaries? Surely there must be a better way to do this … let’s see what other people on the net have done! Oh right … they split text into “interface” and “per scene” libraries, which doesn’t work for a game like DD. So let’s see if we can pull text from a file only as we need it. First thing we need is to do is move the text from the TextManager to an external file (this is a good idea for localisation anyway, so not a bad time to do it). You make every dictionary entry a new line in the text file, so far so good.
Then you look at some text in the game … and instead of new lines, you see \n everywhere.
So some background on characters: When defining strings in code, a backslash is a special character. The compiler reads it and the next character, and does something special with it. This is where \n comes in, since it means ‘make a new line’. As far as ASCII is concerned, \n is one character, not two.
So now you go back to your newly minted text file. When you read in \n from the file, what you’re actually reading in, is two characters: \\ and n. You’d think the way to solve this would be to go through the file replacing the \\ with \ … but you can’t, because \ on its own is not a valid character. Once again, we turn to the internet to help us. Surely someone else has figured out a way to solve this! Nope … every solution is either naive, or does a custom job of inserting all known special characters when it comes across the \\ + special char combo.
Eventually you realise that most of the special characters can be saved correctly in the text file without breaking your importer (things like tabs and quotation marks), all it takes is a few good ‘search and replace’ calls. Except \n. If you were to store a new line as a real new line, you wouldn’t know whether you’d gotten to the end of an entry, or just a new line in the text block. In the end it’s ok, you just code it to replace \\n with \n while importing, and move onto something else, because this text stuff has already take up way too much time …